Day 12: Strings

Welcome back fellow Rustaceans π¦
In the last article, we learned how different languages move around variables allocated on heap memory. Then we looked at how this memory is allocated and freed in different languages. Before we look at how all of this is done in Rust, we will look at our first heap-allocated type in Rust: Strings. Strings are like dynamic arrays of chars. They can grow and shrink in size, and they offer a variety of methods for manipulation.
In Rust, we use the String
type to represent strings. To create a string in Rust, you can use the from
method of the String
type from the Rust standard library. When we call this method, the Rust library internally allocates memory on the heap and initializes it with the content passed to the from
method.
let mut my_string = String::from("Rustπ¦");
One of the things you will notice is that we can have emojis as part of strings. So awesome, right? Well, if you remember what we learned in day 5 of this series, Rust's char
type is not limited to a single byte. Instead, it represents a single Unicode Scalar Value. Similar to Rust char
s, Rust strings are not limited to ASCII chars and can represent UTF-8. This ensures your Rust programs can handle text from around the world!
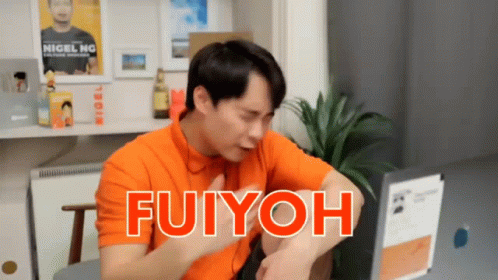
Now let's try to access the first element of the string. From what we know in C, strings can be thought of as arrays of char
and can be accessed using indexes. Let's try that in Rust:
let mut message = String::from("Rustπ¦");
println!("First elemnt of message is {}", message[0]);
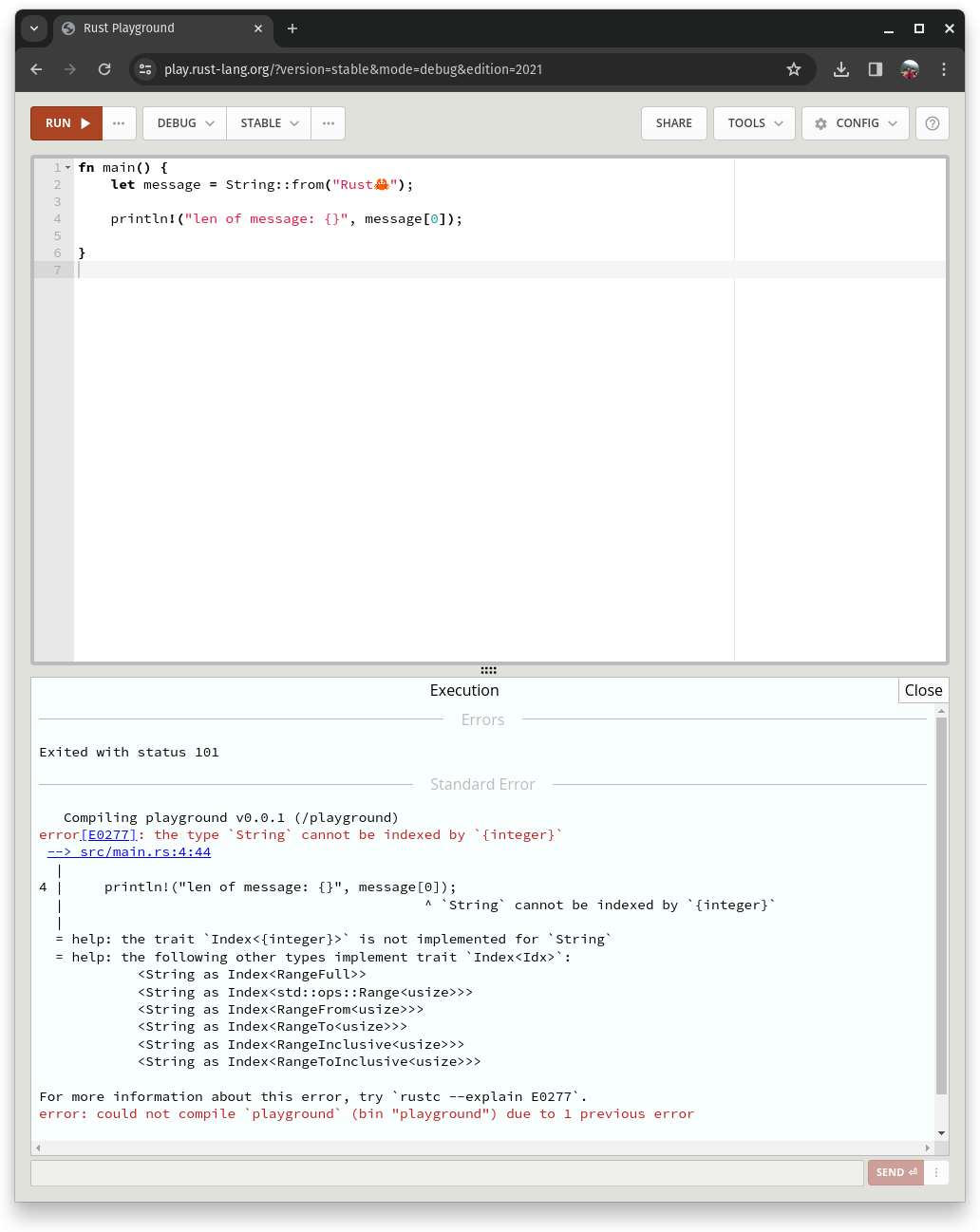
Okay, the Rust compiler is not Happy again, Can you guess why?